Essential Numpy Interview Questions for Data Science & Python Developers
Ace your next interview with our guide on Numpy interview questions. Explore 50 essential questions, from beginner to advanced, covering array operations, broadcasting, and linear algebra.
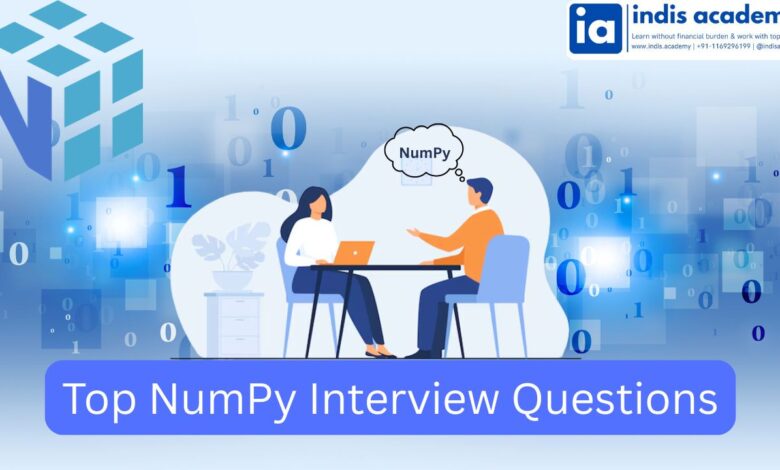
Advanced-Level Questions (36-50)
36. What is the np.einsum()
function and when would you use it?
Answer:
The np.einsum()
function provides a way to express complex array manipulations, such as matrix products, transpositions, and other tensor operations, using a concise and readable notation. It’s often used for tasks involving summation of products, inner products, outer products, etc.
It can be significantly more efficient than traditional matrix multiplication functions, especially when working with large datasets.
Example:
37. How does Numpy optimize memory usage when creating large arrays?
Answer:
Numpy optimizes memory usage through several techniques, such as:
- Memory view: Instead of copying data, Numpy allows for creating a view on the original data using
np.view()
. This saves memory because the data is not duplicated. - Data type optimization: Numpy allows you to specify the data type (
dtype
) of arrays, which can help reduce memory usage. For instance, usingnp.float32
instead ofnp.float64
can save memory in large arrays. - Contiguous memory allocation: Numpy stores arrays in a contiguous block of memory, which reduces overhead compared to Python lists, which can be scattered.
38. How would you perform polynomial fitting using Numpy?
Answer:
Polynomial fitting can be performed using np.polyfit()
, which fits a polynomial of a specified degree to a set of data points using the least squares method. This is commonly used for regression tasks.
Example:
39. Explain how the np.linalg.svd()
function works in linear algebra.
Answer:
The np.linalg.svd()
function computes the Singular Value Decomposition (SVD) of a matrix. SVD is a method of decomposing a matrix into three other matrices: U
, S
, and V
such that:
Where:
U
is an orthogonal matrix (left singular vectors),S
is a diagonal matrix of singular values,V.T
is the transpose of the orthogonal matrix (right singular vectors).
This decomposition is fundamental in tasks like dimensionality reduction (e.g., PCA), solving linear systems, and more.
40. How can you handle missing values in Numpy arrays?
Answer:
In Numpy, missing data is often represented as np.nan
(Not a Number). You can handle missing values using:
np.isnan()
: To check for NaN values.np.nanmean()
: To calculate the mean while ignoring NaN values.np.nan_to_num()
: To replace NaN with a specific value (like 0).
Example:
41. How would you compute the covariance matrix of two Numpy arrays?
Answer:
You can compute the covariance matrix using np.cov()
, which returns the covariance matrix between two or more variables. For example:
42. What is the np.tile()
function used for?
Answer:
np.tile()
is used to repeat an array a specified number of times along each axis. It’s useful when you need to replicate data to create larger arrays.
Example:
43. What are some common optimizations you can use with Numpy for large datasets?
Answer:
- Use appropriate data types: Choosing a smaller
dtype
, such asnp.float32
instead ofnp.float64
, can reduce memory usage. - Vectorization: Avoid loops by using Numpy’s vectorized operations, which are faster and more memory-efficient.
- In-place operations: Modify arrays in place (e.g., using
+=
,-=
) to avoid creating unnecessary copies of data. - Memory mapping: Use
np.memmap()
for memory-mapped arrays to efficiently work with large datasets that don’t fit in memory.
44. What is the difference between np.nanmean()
and np.mean()
?
Answer:
np.mean()
calculates the mean of an array, but it does not handle NaN values. If the array contains NaN values, the result will also be NaN. In contrast, np.nanmean()
ignores NaN values and calculates the mean based only on the non-NaN elements.
45. How can you use Numpy for advanced image processing tasks?
Answer:
Numpy is frequently used in image processing because images can be represented as multi-dimensional arrays (e.g., 2D for grayscale or 3D for RGB images). You can use Numpy for tasks like:
- Reshaping and resizing images: Use
np.reshape()
andnp.resize()
. - Filtering images: Apply filters using convolution (e.g., with
np.convolve()
). - Image transformations: Rotate, flip, or apply geometric transformations to image data arrays.
46. How do you compute eigenvalues and eigenvectors using Numpy?
Answer:
You can compute the eigenvalues and eigenvectors of a square matrix using np.linalg.eig()
. It returns two arrays: the first contains the eigenvalues, and the second contains the corresponding eigenvectors.
47. What are the advantages of using Numpy over Python’s built-in list for scientific computing?
Answer:
- Performance: Numpy arrays are implemented in C and optimized for speed, especially for mathematical operations, whereas Python lists are slower and less memory-efficient.
- Functionality: Numpy provides a wide range of mathematical and statistical functions, while Python lists do not have these capabilities.
- Memory efficiency: Numpy arrays use less memory and store data more compactly than lists.
48. Explain the np.meshgrid()
function and its use case.
Answer:
np.meshgrid()
is used to create a grid of coordinates, commonly used in 3D plotting or when working with functions of two variables. It takes two 1D arrays and returns two 2D arrays representing the coordinate grid.
Example:
This creates coordinate matrices for 3D plotting or surface calculations.
49. How can you use Numpy in conjunction with Pandas for data analysis?
Answer:
Numpy and Pandas are often used together for data analysis tasks. Pandas is built on top of Numpy, and Numpy arrays are used within Pandas DataFrames. Commonly, you’ll use Numpy to perform fast numerical computations and Pandas to handle data structures like Series and DataFrames.
Example:
50. What are the best practices for optimizing Numpy code in terms of speed and memory efficiency?
Answer:
- Use vectorized operations: Avoid using loops for element-wise operations and rely on Numpy’s vectorized functions.
- Choose appropriate data types: Use smaller
dtype
values when possible to save memory. - In-place operations: Modify arrays in place to reduce memory overhead.
- Use
np.einsum()
for complex operations: When dealing with complex array manipulations,np.einsum()
can be faster and more memory-efficient than traditional methods.
Conclusion:
We’ve covered the top 50 Numpy interview questions, spanning beginner to advanced levels. Mastering these questions and understanding the key Numpy concepts will equip you to confidently tackle any Numpy-related interview. Whether you’re aiming for a Python development, data science, or machine learning role, Numpy’s importance cannot be overstated. Keep practicing, dive deeper into the library, and you’ll be well on your way to acing your interview!