Top Verilog Interview Questions With Answers
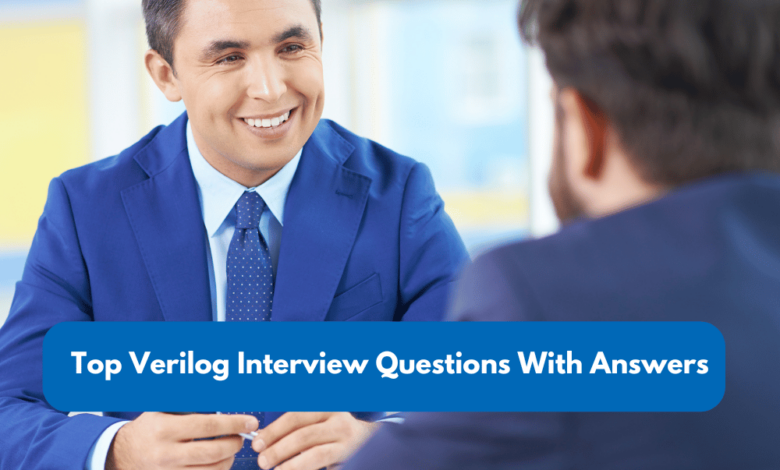
Verilog, a hardware description language (HDL), plays a pivotal role in digital system design and verification. Introduced in the mid-1980s, Verilog has become a cornerstone for designing complex digital systems like microprocessors, memory modules, and application-specific integrated circuits (ASICs). Its ability to model both the structure and behavior of electronic circuits makes it a preferred choice for engineers and organizations worldwide.
Preparing for a Verilog interview requires a strong grasp of theoretical concepts, practical applications, and problem-solving abilities. Whether you’re a fresher stepping into the world of digital design or a seasoned professional aiming for a challenging role, understanding the type of questions commonly asked in Verilog interviews is essential. This guide is designed to help you tackle Verilog interview questions effectively, covering basic, intermediate, and advanced levels.
From foundational concepts like data types and operators to advanced topics such as synthesis constraints and simulation intricacies, this content will equip you with insights to confidently navigate your interview. Let’s dive into some of the most commonly asked Verilog interview questions and how to address them comprehensively.
Top Verilog Interview Questions
Check here Top Verilog Interview Questions which will help you to qualify your interview.
Basic Verilog Interview Questions with Answer:
1. What is Verilog, and why is it used?
Verilog is a Hardware Description Language (HDL) used to model, design, and simulate digital systems. It enables designers to describe the structure and behavior of digital circuits using code, making it easier to test and debug before physical implementation. Verilog is widely used in ASIC and FPGA design because it supports simulation, synthesis, and verification.
2. What are the key data types in Verilog?
Verilog has three primary data types:
- Wire: Represents a physical connection; used for continuous assignment.
- Reg: Used in procedural blocks; stores a value until updated.
- Vector: Represents multiple bits (e.g.,
wire [3:0] data
). Understanding the use of these types is critical for proper circuit modeling.
3. What is the difference between wire
and reg
?
Wire
represents a connection and is used with continuous assignments or module ports. It cannot store values. Reg
stores values and is used in procedural blocks (always
, initial
). For example:
wire signal; // Used for connections reg data; // Used for storage within procedural logic
4. Explain the always
block in Verilog.
The always
block is a procedural construct that executes whenever a specified sensitivity list changes. It is commonly used for sequential or combinational logic. Example:
always @(posedge clk) // Triggered on clock's rising edge q <= d; // Sequential logic
It supports synchronous (posedge
/negedge
) and asynchronous events.
5. What are blocking and non-blocking assignments?
- Blocking (
=
): Executes sequentially, one after another. - Non-blocking (
<=
): Executes concurrently, allowing parallel updates. Example:
// Blocking a = b; // b is assigned to a immediately c = a; // Non-blocking a <= b; // Scheduled but not executed immediately c <= a;
Non-blocking assignments are preferred in sequential logic.
6. What is a testbench in Verilog?
A testbench is a Verilog module that provides input stimuli to the Design Under Test (DUT) and observes the outputs. It includes no hardware implementation and is used only for simulation. Example structure:
module testbench; reg clk, reset; wire output_signal; DUT dut_inst(.clk(clk), .reset(reset), .output_signal(output_signal)); initial begin // Apply test vectors end endmodule
7. What is the difference between module
and endmodule
?
module
starts the definition of a module, the building block of Verilog. endmodule
marks the end of the module definition. Example:
module my_module(input wire a, output wire b); assign b = a; endmodule
8. Explain the difference between assign
and always
.
assign
: Used for continuous assignments, primarily for combinational logic.always
: Used for procedural logic, including sequential and combinational logic. Example:
assign sum = a + b; // Continuous assignment always @(a or b) begin sum = a + b; // Procedural assignment end
9. What is the purpose of sensitivity lists in the always
block?
Sensitivity lists ensure that the always
block executes only when specified signals change. For example:
always @(a or b) // Executes when a or b changes c = a + b;
Using always @*
automates sensitivity list generation for combinational logic.
10. What are system tasks in Verilog?
System tasks perform simulation-specific functions. Common examples include:
$display
: Prints text and variables.$finish
: Ends the simulation.$monitor
: Continuously displays variable changes.
11. What is the difference between initial
and always
?
initial
: Executes only once at the start of simulation.always
: Repeatedly executes based on the sensitivity list.
12. How does the case
statement differ from if-else
?
case
is used for multi-way branching, whereas if-else
is suitable for conditional branching. Example:
case (sel) 2'b00: out = a; 2'b01: out = b; default: out = c; endcase
13. What are synthesizable and non-synthesizable constructs in Verilog?
- Synthesizable: Can be converted to hardware (e.g.,
assign
,always
). - Non-synthesizable: Used only in simulation (e.g.,
initial
,$display
).
14. What are the different modeling styles in Verilog?
- Behavioral: Describes functionality (
always
,if-else
). - Dataflow: Uses continuous assignments (
assign
). - Structural: Describes using module instantiations.
15. Explain the difference between combinational and sequential logic.
- Combinational: Outputs depend only on current inputs.
- Sequential: Outputs depend on inputs and previous states.
16. What is the significance of posedge
and negedge
?
posedge
(positive edge) and negedge
(negative edge) detect clock transitions for triggering sequential logic.
17. What are generate statements?
Generate statements create hardware iteratively. Example:
genvar i; generate for (i = 0; i < 4; i = i + 1) begin assign out[i] = in[i]; end endgenerate
18. What are the limitations of Verilog?
- Lacks strong typing compared to VHDL.
- Cannot directly model asynchronous events.
19. What is a parameter in Verilog?
Parameters define constants that can be overridden during module instantiation. Example:
module my_module #(parameter WIDTH = 8) (input [WIDTH-1:0] in); endmodule
20. How does timescale
affect simulation?
The timescale
directive sets the time unit and precision. Example:
`timescale 1ns/1ps
This sets a 1-nanosecond unit with 1-picosecond precision.
21. What are blocking statements used for?
Blocking statements ensure sequential execution, commonly used in testbenches for control flow.
22. How do you declare a memory in Verilog?
Memory is declared as an array. Example:
reg [7:0] mem[0:255]; // 256x8 memory
23. What is a null module?
A module with no functionality, used as a placeholder for testing.
24. Can Verilog model analog circuits?
Verilog primarily models digital circuits. Analog modeling requires Verilog-A or other mixed-signal tools.
25. What are synthesis constraints?
Constraints guide synthesis tools for timing, area, and power optimization.
26. What is the difference between <=
and =
?
<=
is non-blocking (used in sequential logic).=
is blocking (used in combinational logic).
27. How is a flip-flop implemented in Verilog?
Using an always
block with posedge
. Example:
always @(posedge clk) q <= d;
28. What are tasks and functions in Verilog?
- Tasks: Perform multiple statements; allow delays.
- Functions: Return a value; no delays allowed.
29. What is the role of a module
in Verilog?
Modules represent hardware components and are the building blocks of Verilog design.
30. What are race conditions?
Race conditions occur when multiple signals update simultaneously, leading to unpredictable behavior. Proper use of non-blocking
assignments mitigates this issue.
Intermediate Verilog Interview Questions With Answer:
31. Explain the difference between Verilog tasks and functions. Provide examples.
- Tasks: Perform multiple operations and can include delays, events, or simulation-specific constructs. Tasks do not return a value.
- Functions: Perform a single computation and must return a value. Functions cannot include delays or simulation-specific constructs.
Example of Task:
task add_numbers; input [7:0] a, b; output [7:0] sum; begin sum = a + b; // Multiple operations allowed end endtask
Example of Function:
function [7:0] add_numbers; input [7:0] a, b; begin add_numbers = a + b; // Returns a single value end endfunction
Key Differences:
- Tasks are more versatile but less efficient in certain use cases.
- Functions are restricted to computations and improve code modularity.
32. What is always @*
in Verilog, and how does it differ from always @(a or b)
?
always @*
is an implicit sensitivity list that automatically includes all signals used within the block. It is used for modeling combinational logic, ensuring no missing signals in the sensitivity list.
Example:
always @* begin sum = a + b; // Automatically sensitive to a and b end
In contrast, always @(a or b)
explicitly lists the signals to which the block is sensitive. Any missing signal could lead to incorrect simulation behavior.
33. What are Verilog delays (#
), and how are they used?
Delays in Verilog simulate the propagation time of signals. They are used in simulation but are non-synthesizable.
Example:
assign #5 y = a & b; // y is updated 5 time units after a & b change
Types of Delays:
- Inertial Delay: Ignores input changes occurring before the delay period ends.
- Transport Delay: Propagates all input changes.
Delays are useful in testbenches for modeling timing behavior.
34. What is the difference between parameter
and defparam
in Verilog?
arameter
: Declares constants that can be overridden during module instantiation.defparam
: Allows changing parameter values post-instantiation.
Example of Parameter:
module my_module #(parameter WIDTH = 8) (input [WIDTH-1:0] in); endmodule
Example of Defparam:
defparam instance_name.PARAM_NAME = new_value;
Key Differences:
parameter
is preferred for modern designs due to better readability.defparam
is considered outdated and less readable.
35. Explain the concept of blocking and non-blocking assignments with examples.
- Blocking (
=
): Executes sequentially within the same block. - Non-blocking (
<=
): Executes concurrently, scheduling updates for the next time step.
Example of Blocking:
always @(posedge clk) begin a = b; // Immediate update c = a; // c gets the updated value of a end
Example of Non-blocking:
always @(posedge clk) begin a <= b; // Scheduled update c <= a; // c gets the old value of a end
Non-blocking assignments are used in sequential logic to prevent race conditions.
36. What is the difference between a latch and a flip-flop in Verilog?
- Latch: Level-sensitive; triggered by a signal’s level (high or low).
- Flip-flop: Edge-sensitive; triggered by a signal’s edge (rising or falling).
Latch Example:
always @(enable) if (enable) q = d; // Latch behavior
Flip-Flop Example:
always @(posedge clk) q <= d; // Flip-flop behavior
Flip-flops are preferred for synchronous designs, while latches can cause timing issues if not used carefully.
37. What are Verilog macros, and how are they defined?
Macros are preprocessor directives used for code simplification and reuse. They are defined using the define
keyword.
Example:
`define ADD(a, b) a + b wire [7:0] result = `ADD(4, 5); // Expands to 4 + 5
Macros simplify repetitive code but should be used judiciously to maintain readability.
38. What are synthesis directives in Verilog?
Synthesis directives guide synthesis tools for optimizing hardware. Common directives include:
full_case
: Assumes all cases are covered.parallel_case
: Assumes no overlap in case conditions.
Example:
// pragma translate_off // Simulation-only code // pragma translate_on
Directives are tool-specific and not part of the standard Verilog language.
39. How do you model tristate buffers in Verilog?
Tristate buffers are modeled using assign
with conditional operators.
Example:
assign out = enable ? data : 1'bz; // High-impedance when not enabled
Tristate buffers are commonly used for shared data buses.
40. What is the purpose of a generate
block in Verilog?
generate
blocks enable conditional or iterative instantiation of hardware components.
Example:
genvar i; generate for (i = 0; i < 4; i = i + 1) begin assign out[i] = in1[i] & in2[i]; end endgenerate
This approach is efficient for replicating repetitive structures.
41. How do you handle asynchronous reset in Verilog?
An asynchronous reset is handled by including it in the sensitivity list.
Example:
always @(posedge clk or posedge reset) if (reset) q <= 0; // Reset state else q <= d; // Normal operation
42. What are Verilog attributes, and how are they used?
Attributes provide additional metadata to synthesis tools. They are defined using the (* ... *)
syntax.
Example:
(* keep = "true" *) wire signal; // Prevent optimization
43. How does the posedge
and negedge
sensitivity affect design?
posedge
: Detects rising edges.negedge
: Detects falling edges.
These are critical for designing edge-triggered sequential circuits.
44. What is the role of casez
and casex
?
casez
: Treatsz
as a wildcard.casex
: Treats bothz
andx
as wildcards.
Example:
casez (value) 4'b1???: out = 1; // Matches with z or x endcase
45. How do you define multidimensional arrays in Verilog?
reg [7:0] mem[0:3][0:7]; // 2D array
Advanced Verilog Interview Questions With Answer:
46. How is a Finite State Machine (FSM) implemented in Verilog?
An FSM in Verilog typically consists of three parts:
- State Encoding: Define states using
parameter
ortypedef
. - State Transition Logic: Define transitions based on inputs.
- Output Logic: Generate outputs based on the current state.
Example:
module fsm_example( input clk, reset, input in, output reg out ); // State Encoding typedef enum reg [1:0] {IDLE, S1, S2} state_t; state_t state, next_state; // State Transition Logic always @(posedge clk or posedge reset) begin if (reset) state <= IDLE; else state <= next_state; end // Next State Logic always @* begin case (state) IDLE: next_state = in ? S1 : IDLE; S1: next_state = in ? S2 : IDLE; S2: next_state = in ? S2 : S1; default: next_state = IDLE; endcase end // Output Logic always @* begin out = (state == S2); end endmodule
47. What are the differences between case
, casex
, and casez
in Verilog?
case
: Performs exact matching; no wildcards.casex
: Treatsx
andz
as wildcards, allowing partial matches.casez
: Treats onlyz
as a wildcard, notx
.
Example:
casez (value) 4'b1???: out = 1; // Matches 4'b1xxx where x can be 0, 1, or z endcase
Use casex
with caution as it may lead to unintended behavior due to wildcard matching of x
.
48. How can you model a clock divider in Verilog?
A clock divider reduces the frequency of the input clock signal.
Example:
module clock_divider( input clk_in, input reset, output reg clk_out ); reg [31:0] counter; always @(posedge clk_in or posedge reset) begin if (reset) begin counter <= 0; clk_out <= 0; end else begin if (counter == 49999) begin // Assuming 50 MHz clock, divide by 100k clk_out <= ~clk_out; counter <= 0; end else begin counter <= counter + 1; end end end endmodule
49. Explain how Verilog handles multiple drivers for a signal.
When multiple drivers assign a value to the same signal, Verilog uses a resolution function to determine the final value:
- If all drivers assign the same value, that value is used.
- If conflicting values are assigned, the signal becomes
x
(unknown).
Example:
assign signal = enable1 ? data1 : 1'bz; // Driver 1 assign signal = enable2 ? data2 : 1'bz; // Driver 2
In the above case, if both enable1
and enable2
are high simultaneously, signal
may resolve to x
.
50. How do you handle parameterized modules in Verilog?
Parameterized modules allow flexible designs that can be reused with different configurations.
Example:
module adder #(parameter WIDTH = 8) ( input [WIDTH-1:0] a, b, output [WIDTH:0] sum ); assign sum = a + b; endmodule // Instantiation with parameter override adder #(16) adder_instance (.a(a), .b(b), .sum(sum));
51. How is a dual-port RAM modeled in Verilog?
Dual-port RAM allows simultaneous access to two memory locations.
module dual_port_ram ( input clk, input we1, we2, input [3:0] addr1, addr2, input [7:0] data_in1, data_in2, output reg [7:0] data_out1, data_out2 ); reg [7:0] mem [15:0]; // 16x8 memory always @(posedge clk) begin if (we1) mem[addr1] <= data_in1; else data_out1 <= mem[addr1]; if (we2) mem[addr2] <= data_in2; else data_out2 <= mem[addr2]; end endmodule
52. What are system functions in Verilog, and provide some examples?
System functions start with $
and are used for simulation and debugging. Examples include:
$display
: Prints messages during simulation.$time
: Returns the current simulation time.$random
: Generates random numbers.
initial begin $display("Simulation started at time: %t", $time); $finish; end
53. How do you prevent latches in Verilog designs?
Latches occur when combinational logic is incomplete or when conditions are not fully specified.
Example of Latch-Prone Code:
always @* begin if (enable) out = data; // Latch inferred if `enable` is false end
Fix:
always @* begin out = 0; // Default value prevents latch if (enable) out = data; end
54. What is the difference between $monitor
, $display
, and $strobe
?
$display
: Prints values immediately when encountered.$strobe
: Prints values at the end of the simulation time step.$monitor
: Continuously tracks and prints values whenever they change.
initial begin $monitor("Time=%t, a=%b, b=%b", $time, a, b); end
55. How can you implement a parameterized N-bit shift register in Verilog?
module shift_register #(parameter WIDTH = 8) ( input clk, reset, input [WIDTH-1:0] data_in, output reg [WIDTH-1:0] data_out ); always @(posedge clk or posedge reset) begin if (reset) data_out <= 0; else data_out <= {data_out[WIDTH-2:0], data_in[WIDTH-1]}; end endmodule
This code defines a shift register where WIDTH
can be configured during instantiation.
Tips for Verilog Interviews:
Preparing for a Verilog interview requires more than just theoretical knowledge. A strong grasp of concepts, practical experience, and problem-solving abilities are crucial for success. Here are some essential tips to help you excel in your Verilog interview:
Understand the Basics: Revise core concepts such as always
blocks, assign
statements, data types (wire
, reg
), and modeling styles (behavioral, dataflow, and structural). A solid foundation will help you answer technical questions confidently.
Master Sequential and Combinational Logic: Be prepared to design flip-flops, latches, multiplexers, and state machines. Understanding the difference between blocking and non-blocking assignments is essential for avoiding race conditions.
Practice Writing Code: Many interviews include practical coding assignments. Practice writing Verilog modules, testbenches, and synthesizable designs to demonstrate your skills.
Learn Debugging Techniques: Be familiar with simulation tools and debugging techniques using $display
, $monitor
, and $strobe
.
Showcase Problem-Solving Skills: Focus on optimizing designs for area, timing, and power efficiency. Demonstrating real-world applications of Verilog in FPGA or ASIC projects can leave a strong impression.
Stay Updated: Familiarize yourself with advanced concepts like clock-domain crossing, parameterized modules, and constraints for synthesis.
Conclusion:
Acing a Verilog interview requires mastering the fundamentals, practical coding skills, and a problem-solving mindset. Revisit core concepts like data types, modeling styles, and constructs (always
, assign
, and testbenches). Strengthen your understanding of sequential and combinational logic by practicing circuits such as multiplexers, flip-flops, and FSMs.
Hands-on coding is essential—practice writing efficient and synthesizable Verilog modules, debugging testbenches, and optimizing designs for real-world applications. Highlight your experience with FPGA or ASIC projects to showcase your practical knowledge and ability to handle complex challenges. Familiarity with simulation tools and system tasks like $monitor
and $display
is crucial for debugging and verification.
Staying updated on advanced topics like clock-domain crossing, generate blocks, and synthesis constraints will give you a competitive edge. Approach challenging questions logically, and clearly articulate your thought process to stand out as a confident and competent candidate.
In summary, preparation is key. By combining technical expertise, practical experience, and effective communication, you can excel in your Verilog interview and take a significant step forward in your hardware design career.